استفاده از ویرایشگر tinymce در php و mysql
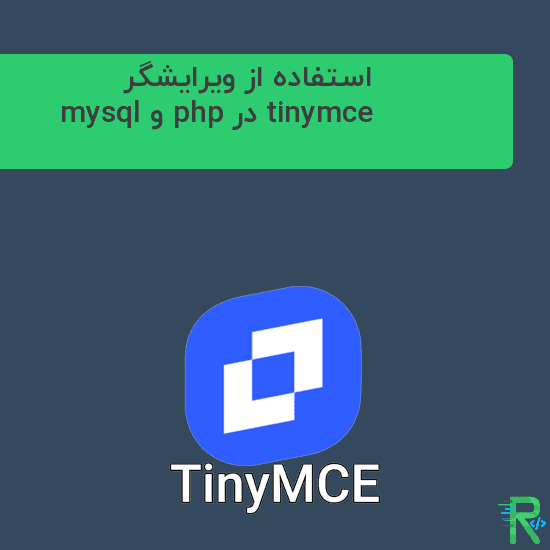
tinymce در php – در این آموزش با استفاده از html , css , js , php , mysql یک cms ساده را می سازیم که ویرایش محتوای سایت را با ویرایشگر قدرتمند tinymce ویرایش می کنیم .
تشریح اسکریپت ها :
- create.php : فرم ایجاد مطلب
- edit.php : فرم ویرایش مطلب
- process.php : پردازش اطلاعات وارده از فرم ها
- list.php : لیست مطالب ایجاد شده
- view.php : نمایش اطلاعات کامل مطلب
- functions.php : توابع مورد نیاز جهت مدیریت ویژگی های برنامه
- app.js : پیکربندی tinymce در جاوا اسکریپت
ویدیوی خروجی برنامه tinymce
1- اسکریپت create.php
<?php require_once "parts/dashboard/header.php";?> <?php show_form_error(true);?> <form action="process.php" method="post"> <input type="text" name="title" id="title" placeholder="عنوان" value="<?=form_value('title')?>"><br> <textarea name="content" id="content" placeholder="توضیحات"><?=form_value('content')?></textarea> <input type="hidden" name="ref" id="ref" value="<?=filename_to_ref(__FILE__)?>"> <input type="submit" name="save" id="save" value="ثبت"> </form> <?php require_once "parts/dashboard/footer.php";?>
2- اسکریپت edit.php
<?php require_once "parts/dashboard/header.php";?> <?php show_form_error(true);?> <?php $row = $GLOBALS['data_middleware_callback'];?> <form action="process.php" method="post"> <input type="text" name="title" id="title" placeholder="عنوان" value="<?=form_value('title', $row['title'])?>"><br> <textarea name="content" id="content" placeholder="توضیحات"><?=form_value('content', $row['content'])?></textarea> <input type="hidden" name="ref" id="ref" value="<?=filename_to_ref(__FILE__)?>"> <input type="hidden" name="id" id="id" value="<?=$_GET['id']?>"> <input type="submit" name="save" id="save" value="ثبت"> </form> <?php require_once "parts/dashboard/footer.php";?>
3- اسکریپت process.php
<?php require_once __DIR__ . "/inc/functions.php"; if (isset($_POST['ref'])) { $ref = $_POST['ref']; $body = $_POST; $method = "post"; } else if (isset($_GET['ref'])) { $ref = $_GET['ref']; $body = $_GET; $method = "get"; } $callback = generate_form_handler_callback($method, $ref); if (is_callable($callback)) { $callback($body); } else { die("callback {$callback} not found"); } ?>
4- اسکریپت list.php
<?php require_once "parts/dashboard/header.php";?> <h1>لیست مطالب</h1> <?php $rows = $GLOBALS['data_middleware_callback']; if (isset($rows['id'])) { $rows = [$rows]; } if ($rows): foreach ($rows as $row): ?> <div class="col-4 the-content mt-2"> <h2><?=$row['title']?></h2> <div class="actions"> <a href="edit.php?id=<?=$row['id']?>" class="text-decoration-none" id="edit-content" title="ویرایش" target="_blank">✎</a> </div> <div class="excerpt"><?=getContentExcerpt($row['content'], 30)?></div> <a class="more-content" href="<?=getViewLinkPost($row['id'])?>" target="_blank">بیشتر</a> </div> <?php endforeach; else: echo get_message_html_template("مطلبی وجود ندارد"); endif; ?> <?php require_once "parts/dashboard/footer.php";?>
5- اسکریپت view.php
<?php require_once "parts/dashboard/header.php";?> <?php $row = $GLOBALS['data_middleware_callback'];?> <a href="list.php">لیست مطالب</a> <h1><?=$row['title']?></h1> <div class="body"><?=$row['content']?></div> <?php require_once "parts/dashboard/footer.php";?>
6- اسکریپت functions.php
<?php require_once "db.php"; require_once "constants.php"; require_once "main.php"; function add_to_root_url($path) { $final_url = ROOT_URL . "/{$path}"; return $final_url; } function check_old_url_to_cookie() { setcookie("old_url", CURRENT_URL, 0); if (!isset($_COOKIE['old_url'])) { $_COOKIE['old_url'] = "/"; } } function check_form_validation_error() { if (($_COOKIE['form_validation_error'] ?? "") != "") { $GLOBALS['form_validation_error'] = $_COOKIE['form_validation_error']; setcookie('form_validation_error', false, (time() - 10)); } } function check_form_old_value() { if (($_COOKIE['form_validation_old_value'] ?? "") != "") { $GLOBALS['form_validation_old_value'] = json_decode($_COOKIE['form_validation_old_value'], true); setcookie('form_validation_old_value', false, (time() - 10)); } } function check_middleware_callback() { $current_script_name = $_SERVER['SCRIPT_NAME']; $ref = filename_to_ref($current_script_name); $callback = generate_middleware_callback($ref); if (is_callable($callback)) { $res = $callback(); $GLOBALS['data_middleware_callback'] = $res; } } function get_message_html_template($text = null) { $str = "<p class=\"bg-danger text-dark\">x-text</p>"; if ($text) { $str = str_replace(['x-text'], [$text], $str); } return $str; } function show_form_error($echo = false, $template = "") { $str = ""; $template = $template ?: get_message_html_template(); if ($GLOBALS['form_validation_error'] ?? false) { $template = str_replace(['x-text'], [$GLOBALS['form_validation_error']], $template); $str = trim($template); } if ($str != "" && $echo) { echo $str; } return $str; } function form_value($key, $default = "") { return $GLOBALS['form_validation_old_value'][$key] ?? $default; } function the_list_of_form_fields() { return [ "create" => [ "title" => [], "content" => [], ], "edit" => [ "id" => [], ], ]; } function get_page_link_by_name($page_name) { $list = the_list_of_form_fields(); $page_link = ""; $filename = $list[$page_name] ?? false; if ($filename) { $the_filename = ref_to_filename($page_name); $page_link = "/{$the_filename}"; } return $page_link; } function generate_form_handler_callback($method, $ref) { return "{$method}_{$ref}_handler"; } function generate_middleware_callback($ref) { return "{$ref}_action_middleware"; } function filename_to_ref($filename) { $the_filename = basename($filename); $the_filename_list = explode(".", $the_filename); $the_filename = $the_filename_list[0]; return $the_filename; } function ref_to_filename($ref) { $filename = "{$ref}.php"; return $filename; } function directTo($address) { header("Location: {$address}"); exit(); } function directToBack() { directTo($_COOKIE['old_url']); } function getViewLinkPost($id) { return add_to_root_url("view.php?id={$id}"); } function get_required_form_fields($ref) { $list = the_list_of_form_fields(); $required_list = $list[$ref] ?? false; if (!$required_list) { die("no required list for {$ref}"); } return $required_list; } function handle_required_list(&$body, $list, $autoTriggerValidation = true) { $the_list = array_keys($list); $empty_list = []; foreach ($the_list as $the_key) { $value = remove2OrMoreWhitespace($body[$the_key] ?? ""); if ($value == "") { $empty_list[] = $the_key; } else { $body[$the_key] = $value; } } if ($empty_list && $autoTriggerValidation) { $validation_message = join(",", $empty_list) . " خالی می باشد"; setcookie("form_validation_error", $validation_message, 0); setcookie("form_validation_old_value", json_encode($body), 0); $filename = ref_to_filename($body['ref']); directToBack(); } return $empty_list; } function get_edit_link($id) { $link = get_page_link_by_name("edit") . "?id={$id}"; return $link; } function checkForRecordOr404($table, $data = [], $whereQuery = [], $message = "Not Found") { $row = selectRow($table, $data, $whereQuery); if (!$row) { die($message); } return $row; } function remove2OrMoreWhitespace($value) { $result = trim(preg_replace("/\s{2,}/i", " ", $value)); return $result; } function merge_second_to_first_if_valid($first, $second, $check_list, $baseCheckValid) { foreach ($check_list as $element) { $the_value = remove2OrMoreWhitespace($second[$element] ?? ""); $res = $baseCheckValid($the_value); if ($res) { $first[$element] = $the_value; } } return $first; } function getContentExcerpt(string $str, int $word = 5) { $tmpStr = trim($str); $word = abs($word); if ($tmpStr == "") { return $str; } $list = explode(" ", $tmpStr); if (count($list) < $word) { $word = count($list); } $tmpStr = array_slice($list, 0, $word); $tmpStr = join(" ", $tmpStr); return $tmpStr; } function abortIfEntityIsInvalid($key, $callback = null) { $entity = $_GET[$key] ?? false; $callback = $callback ?: function ($item) { return $item; }; if (!$callback($entity)) { die("{$key} is invalid or empty"); } return $entity; } #=========> FORM HANDLERS function post_create_handler($body) { handle_required_list($body, get_required_form_fields($body['ref'])); $id = insertRow([ "title" => $body['title'], "content" => $body['content'], ], "tinymce"); directTo(get_edit_link($id)); } function post_edit_handler($body) { handle_required_list($body, get_required_form_fields($body['ref'])); $id = $body['id']; $row = checkForRecordOr404("tinymce", [$id], "id=?"); $check_list = array_keys(get_required_form_fields("create")); $row = merge_second_to_first_if_valid($row, $body, $check_list, function ($element) { return $element != ""; }); updateRow([ "title" => $row['title'], "content" => $row['content'], ], "tinymce", ["keys" => "id=?", "values" => [$id]]); directToBack(); } #=========> END FORM HANDLERS #=========> MIDDLEWARE CALLBACK function edit_action_middleware() { $id = abortIfEntityIsInvalid("id"); $row = checkForRecordOr404("tinymce", [$id], "id=?"); return $row; } function list_action_middleware() { $row = selectRow("tinymce"); return $row; } function view_action_middleware() { $id = abortIfEntityIsInvalid("id"); $row = checkForRecordOr404("tinymce", [$id], "id=?"); return $row; } #=========> END MIDDLEWARE CALLBACK ?>
7- اسکریپت app.js
window.addEventListener("load" , function(){ tinymce.init({ selector: 'textarea#content', directionality : "rtl", plugins: [ 'a11ychecker','directionality','advlist','advcode','advtable','autolink','checklist','export', 'lists','link','image','charmap','preview','anchor','searchreplace','visualblocks', 'powerpaste','fullscreen','formatpainter','insertdatetime','media','table','help','wordcount' ], toolbar: 'undo redo | ltr rtl | a11ycheck casechange blocks | bold italic backcolor | alignleft aligncenter alignright alignjustify |' + 'bullist numlist checklist outdent indent | removeformat | code table help', language: 'fa' }).then(function(tmce){ }); });
قبل از استفاده از پروژه وارد inc/db.php شده و اطلاعات mysql را وارد کنید سپس وارد پوشه import database شده و دیتابیس را وارد کنید .
لیست نظرات
Warning: Undefined array key "REQUEST_SCHEME" in C:\Users\mahdi\Downloads\tinymce_php-mysql-js\inc\constants.php on line 3 سلام دوست عزیز وقتی پرژه را ران میکنم یک همچین قطعه اروری به من میده مشکل چیه ؟
درود ، باید Apache 2.4 به بعد داشته باشید تا این داده در لیست اعضای سرور قرار بگیره .