افزودن مقایسه محصولات فروشگاه با js و PHP
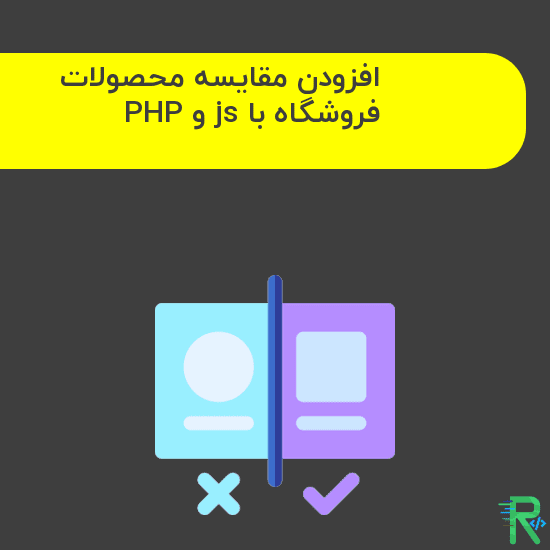
افزودن ویژگی مقایسه محصولات فروشگاه – با کمک ویژگی مقایسه به کاربر این امکان داده می شود که بتواند تصمیم گیری بهتری را برای خرید یک محصول داشته باشد .
روند کار مقایسه محصول این است که ویژگی های محصول را به نمایش می گذارد تا کاربر بتواند مشاهده کند و خرید خود را نهایی کند .
فایل index.php صفحه اصلی مقایسه و نمایش محصول
<!DOCTYPE html> <html lang="fa"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>RapidCode.IR - مقایسه محصولات با PHP , js</title> <link rel="stylesheet" href="static/css/style.css"> </head> <body> <a id="introduce" target="_blank" href="https://rapidcode.ir">رپید کد - کتابخانه مجازی برنامه نویسان</a> <div class="container"> <div class="compare">?</div> <section class="compare-page"> <div class="container-compare"></div> <button id="close-compare-page">خروج</button> </section> <?php require_once "shop.php"; $rows = loop_product(); ?> <h1><?php echo $GLOBALS['title'] ?></h1> <?php foreach ($rows as $row) : ?> <article> <img src="<?php echo $row['thumbnail'] ?>" alt="<?php echo $row['title'] ?>"> <h2><?php echo $row['title'] ?></h2> <p><?php echo $row['content'] ?></p> <span id="price"><?php echo $row['price'] ?> تومان</span><br> <a class="compare-product" id="pid_<?php echo $row['id'] ?>" data-ID="<?php echo $row['id'] ?>">مقایسه</a> </article> <?php endforeach; ?> </div> <script src="static/js/app.js"></script> </body> </html>
فایل shop.php که شامل توابع کاربردی برای دریافت اطلاعات از دیتابیس و … می باشد .
فایل shop.php
<?php function loop_product($pid = "", $select = "*") { $mysqli = new mysqli("localhost", "root", "", "posts"); $mysqli->set_charset("utf8"); $stmt = $mysqli->stmt_init(); $GLOBALS['title'] = "لیست محصولات "; $loop_shop_query = ""; if ($pid) { $loop_shop_query = "WHERE id=?"; } $query = "SELECT {$select} FROM `products` {$loop_shop_query}"; $stmt->prepare($query); if ($pid) { $stmt->bind_param("i", $pid); } $rows = []; if ($stmt->execute() && $res = $stmt->get_result()) { if ($stmt->affected_rows || $res->num_rows) { while ($row_loop = $res->fetch_assoc()) { $rows[] = $row_loop; } } } $stmt->close(); $mysqli->close(); return $rows; } function product_exist($pid) { $mysqli = new mysqli("localhost", "root", "", "posts"); $mysqli->set_charset("utf8"); $stmt = $mysqli->stmt_init(); $query = "SELECT id FROM `products` WHERE id=?"; $is_found = 0; $stmt->prepare($query); $stmt->bind_param("i", $pid); if ($stmt->execute() && $stmt->store_result()) { $is_found = $stmt->affected_rows; $stmt->free_result(); } return $is_found; } ?>
فایل compare.php یک api ساده برای مان فراهم می کند که بتوانیم داده های محصول را به صورت json دریافت کنیم .
فایل compare.php
<?php header("Content-Type: application/json"); require_once "shop.php"; $response_list = [ "status" => 0, "msg" => "", "data" => [] ]; if(empty($_GET['pid'])){ $response_list['msg'] = "آیدی محصول وارد نشده"; die(json_encode($response_list)); }else if(!is_numeric($_GET['pid'])){ $response_list['msg'] = "آیدی محصول معتبر نمی باشد"; die(json_encode($response_list)); }else{ $found = product_exist($_GET['pid']); if (!$found) { $response_list['msg'] = "محصول مورد نظر یافت نشد"; die(json_encode($response_list)); } $row = loop_product($_GET['pid']); $row = end($row); $features = $row['features']; $response_list['data'] = [ "id" => $row['id'], "title" => $row['title'], "thumbnail" => $row['thumbnail'], "price" => $row['price'], "features" => $features, ]; $response_list['status'] = 1; $response_list['msg'] = "موفق !"; echo json_encode($response_list); } ?>
فایل app.js برای ثبت event ها و تعامل با compare.php
فایل app.js
document.addEventListener("DOMContentLoaded", function() { // Global variable window.compareListId = []; // access DOM const compareItemButtonDOM = document.getElementsByClassName("compare-product"); const containerCompareDOM = document.getElementsByClassName("container-compare")[0]; const compareButtonDOM = document.getElementsByClassName("compare")[0]; const compareCanvasDOM = document.getElementsByClassName("compare-page")[0]; const compareCanvasCloseDOM = document.getElementById("close-compare-page"); // other property let requestLock = false; let compareCounter = 0; for(var i=0;i<compareItemButtonDOM.length;i++){ const currentElement = compareItemButtonDOM[i]; currentElement.addEventListener("click" , handlerCompareProduct); } compareButtonDOM.addEventListener("click" , handlerCompareButton); compareCanvasCloseDOM.addEventListener("click" , handlerCloseComparePage); // Handlers function handlerCompareProduct(event){ const thisElement = event.target; const productID = thisElement.getAttribute("data-id"); if(thisElement.getAttribute("compare-status") == "true"){ for(var i=0;i<window.compareListId.length;i++){ const currentIndex = window.compareListId[i]; if(productID == currentIndex){ window.compareListId.splice(i , 1); break; } } thisElement.setAttribute("compare-status" , "false"); thisElement.removeAttribute("style"); changeCompareButtonText(); return; } window.compareListId.push(productID); thisElement.setAttribute("compare-status" , "true"); thisElement.style.background = "tomato"; changeCompareButtonText(); return } function handlerCompareButton(event){ compareCanvasDOM.classList.add("page-active"); if(0 < window.compareListId.length && window.compareListId[compareCounter]){ customGetRequestFunc({ params : { "pid" : window.compareListId[compareCounter] }, loadFunc : function(){ requestLock = false; const response = this.response; if(response.status == 0){ alert(response.msg); return 0; } const data = response.data; const htmlContent = generateHtmlElementFunc(data); containerCompareDOM.innerHTML += htmlContent; //window.compareListId.shift(); compareCounter++; handlerCompareButton(); }, }); }else if(containerCompareDOM.innerHTML == ""){ alert("موردی یافت نشد"); } } function handlerCloseComparePage(event){ const thisElement = event.target; containerCompareDOM.innerHTML = ""; thisElement.parentElement.classList.remove("page-active"); compareCounter = 0; } // ajax request function customGetRequestFunc(options) { if(requestLock == true) return; const xhr = new XMLHttpRequest(); xhr.responseType = "json"; requestLock = true; let queryString = new URLSearchParams; const params = options.params; for (const param in params) { queryString.append(param, params[param]); } queryString = "?" + queryString.toString(); xhr.open("GET", location.href + "/compare.php" + queryString); xhr.onload = options.loadFunc; xhr.onerror = function() { requestLock = false; console.warn("[XHR Error]"); } xhr.send(); } // other function getFeatureKeys(){ return { "dimension" : "ابعاد", "weight" : "وزن" , "specialFeatures" : "ویژگی های خاص", "numSimcard" : "تعداد سیمکارت", "internalStorage" : "حافظه داخلی", "pictureResolution" : "رزولوشن دوربین", "selfieResolution" : "رزولوشن سلفی", "videoRecording" : "فیلمبرداری" }; } function generateHtmlElementFunc(data) { const features = JSON.parse(data.features); const featuresDbKey = Object.keys(features); const featureKeys = getFeatureKeys(); let tableRows = ""; for(var i=0;i<featuresDbKey.length;i++){ let currentKey = featuresDbKey[i]; const currentIndex = features[currentKey]; currentKey = featureKeys[currentKey]; tableRows += `<tr><td>${currentKey}</td><td>${currentIndex}</td></tr>`; } const templateHtml = `<article id="pid-${data.id}"><img src="${data.thumbnail}" alt="${data.title}"><h2>${data.title}</h2><table>${tableRows}</table><span id="price">${data.price} تومان</span><br></article>`; return templateHtml; } function changeCompareButtonText(){ compareButtonDOM.textContent = window.compareListId.length; compareButtonDOM.textContent = (compareButtonDOM.textContent == "0") ? "?" : compareButtonDOM.textContent; } });
و در آخر فایل main.css برای یک ظاهر بهتر
فایل main.css
body { text-align: center; overflow-x: hidden; } #introduce { color: white; text-decoration: none; font-weight: bold; display: block; width: 100%; padding: 5px 10px; background-color: #4CAF50; text-align: center; font-size: 25px; margin-bottom: 45px; } .container { width: 70%; margin: 15px auto; direction: rtl; } .ds-none { display: none !important; } article { width: 25%; border: 2px solid skyblue; padding: 10px; float: right; margin-left: 15px; margin-bottom: 25px; } article table { border-collapse: collapse; min-height: 480px; } article table tr td { border: 1px solid black; } article img { width: 200px; height: 200px; object-fit: cover; } article h2 { height: 82px; overflow: hidden; } article p { height: 56px; overflow: hidden; float: right; margin: 2px 0 15px 0; } article a { text-decoration: none; font-weight: bold; background-color: #4CAF50; color: white; width: 100%; padding: 10px 0; display: block; } #price { color: orange; font-weight: bold; margin: 3px; display: block; } .compare { position: fixed; left: 15px; bottom: 15px; cursor: pointer; background-color: tomato; color: white; font-weight: bold; border-radius: 50%; padding: 15px; width: 18px; } .compare-page { display: none; width: 100%; height: 100vh; background-color: rgba(255, 255, 255, 0.85); position: fixed; z-index: 15; top: 0; left: 0; } .page-active { display: block; } .container-compare article { margin-top: 20px; } .container-compare article img { width: 100px; height: 100px; } .compare-product { background-color: green; cursor: pointer; }
دموی برنامه ( نتیجه )

قبل از اجرای برنامه وارد پوشه product TABLE to import شده table را import کنید
ارسال نظر